I got the chance to get an early CTP of Visual Studio 2010.
This is the post I use to record my first impressions. There is no order to this post, it is just impressions jotted down as I see them.
We seem to have a new start page:
Following the MS & OSS new approach, one of the samples is Dinner Now using Lucene, which is the first project that I found to test.
TFS is still broken:
I really don't like to see this kind of issues in a source control system. It means that it cannot be trusted.
Looks like we have something new here. On first impression, it looks like we have UML integrated into VS.
I took a look at the generated XML, which is the backing store for the diagrams, and it looks like it should work with source control much better than the usual modeling stuff in visual studio.
Another feature that is very welcome for anyone doing presentations is the use of CTRL+Scroll Wheel for zooming.
We are also promised performance improvements for large files, which is nice. Part of the walkthroughs talk about integrating functionality using MEF, which is good.
Looking at the walkthrough for creating syntax highlighting, tagging and intellisense, it looks like a lot of ceremony still, but it seems significantly easier than before.
WPF - It looks like VS is moving to WPF, although this CTP is still midway.
C# has dynamic variables!
dynamic doc = HtmlPage.Document.AsDynamic();
dynamic win = HtmlPage.Window.AsDynamic();
This was talked about in the MVP Summit, a dynamic object is an object that implements IDynamicObject:
Note that we accept an expression parameter (using Linq expressions) and we return a meta object. Show below.
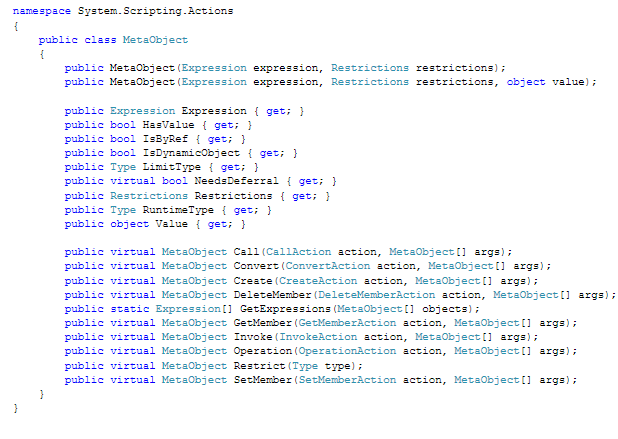
This looks like C# + DLR integration, which is cool. I am looking forward to see what we can do with it.
VS also get some R# like features:
There is also a quick search, apparently, but I am not really impressed. Again, show me something that I don't have.
There is CLR 4.0, so we somehow skipped CLR 3.0. I am glad to know that we have a new runtime version, instead of just patching the 2.0 very slowly.
Threading
System.Threading.Tasks is new, and looks to be very interesting. It also seem to have integration with Visual Studio. It is also interesting because we seem to have a lot more control over that than we traditionally had in the ThreadPoll.
Parallel extensions are also in as part of the framework, not that this would be a big surprise to anyone.
In the CTP that I have, there is nothing about Oslo, models or DSL, which I found disappointing. I guess I'll have to wait a bit more to figure out what is going on.
That was a quick review, and I must admit that I haven't dug deep, but the most important IDE feature, from my perspective, is the CTRL+Scroll wheel zooming. The diagrams support is nice, but I am not sure that I like it in my IDE. Threading enhancements are going to be cool, and I am looking forward to seeing what kind of dynamic meta programming we can do with it.